Hiding the Menu on Right-Click with JavaScript
There are a number of reasons someone would want to disable right-clicks on their websites. Most notably, people do it to prevent users from downloading images, or copying text. Of course, if you’re reading this blog, you probably know how to work around this – there are numerous ways.
Nonetheless, let’s explore how we can accomplish this with JavaScript.
Cancelling the default menu
window.oncontextmenu = () => {
console.log("right click");
return false;
};
The oncontextmenu
event occurs when the user right-clicks on an HTML element to open the context menu. The above function is attaching the oncontextmenu
to the entire window object, thus the function will execute wherever a user right-clicks on the page.
Try it now. Copy the code above and paste it into the browser console.
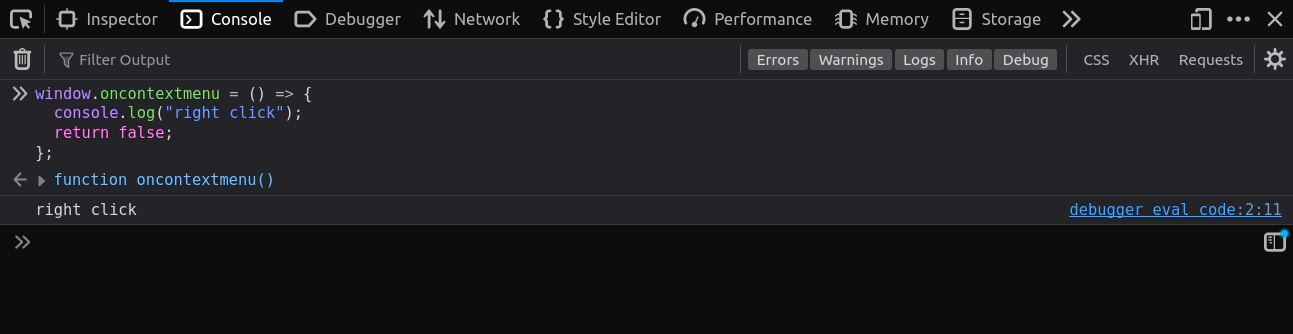
You’ll notice your right-click menu is no longer in use. This is all accomplished by returning false
on the right-click event. You can swap it quickly with true
to have the menu displayed again.
We can also declare a function to accommplish the same:
function handleContextMenu(event) {
console.log("right click");
event.preventDefault();
}
window.addEventListener("contextmenu", handleContextMenu);
After defining the function, we attach an event listener to the window object.
Conclusion
In this article we looked at two seperate ways to hide the context menu on right click events.
Have you ever been on a website where you couldn’t open the menu? Did you find a workaround? Let us know in the comments below.
That wraps it up. We hope you enjoy these bite-sized articles.
Related Posts
Creating a Real Time Chat Application with React, Node, and TailwindCSS
In this tutorial, we will show you how to build a real-time chat application using React and Vite,as well as a simple Node backend.
Read moreThe Importance of Staying Active as a Software Developer
In today’s fast-paced digital world, developers often find themselves glued to their screens for extended periods. While this dedication is commendable, it comes with its own set of challenges.
Read moreJavaScript DOM Mastery: Top Interview Questions Explained
Mastering the Document Object Model (DOM) is crucial for any JavaScript developer. While many developers rely heavily on front-end frameworks, the underlying DOM concepts are still important.
Read more