JavaScript DOM Mastery: Top Interview Questions Explained
Mastering the Document Object Model (DOM) is crucial for any JavaScript developer. While many developers rely heavily on front-end frameworks, the underlying DOM concepts are still important. You might find yourself stumped in an interview if you’re not well-versed in these fundamentals.
This article aims to prepare you for DOM-related interview questions, which can arise even in React interviews. Let’s dive in and ensure you’re ready for anything that comes your way.
Question 1: What is the DOM?
Answer: The Document Object Model (DOM) is a programming interface that allows developers to interact with and manipulate HTML and XML documents. It represents the document as a structured tree of nodes, where each node corresponds to a part of the document (like elements, attributes, or text).
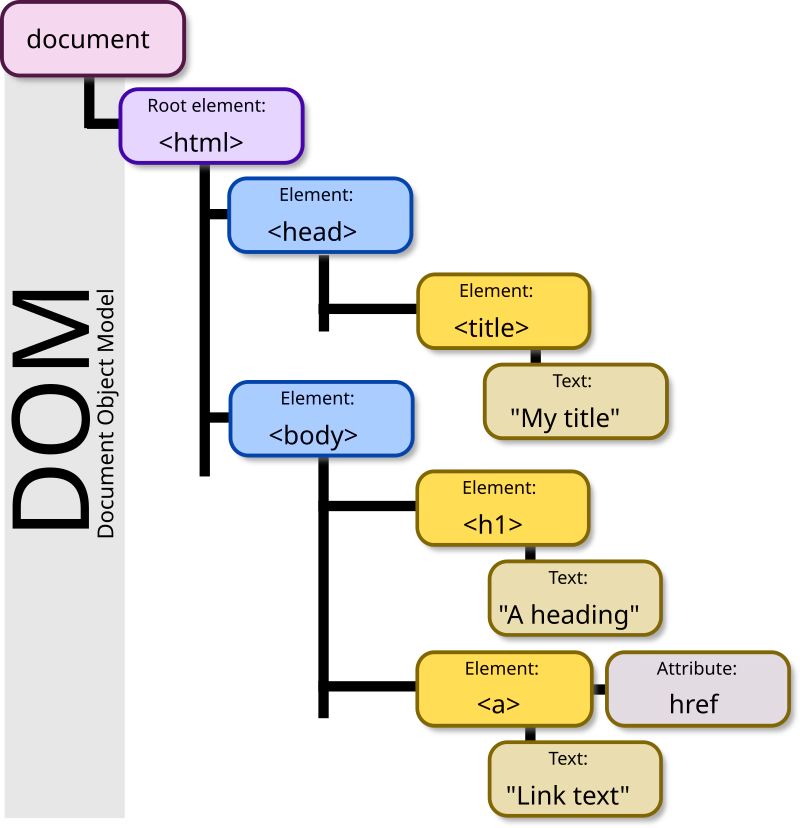
Image source: Wikipedia
Explanation: Think of the DOM as a bridge between your web page and your scripts. By manipulating the DOM, you can dynamically update content, the structure, and the styles of your web page.
Question 2: How do you select an element by its ID in the DOM?
Answer: You can select an element by its ID using the getElementById
method.
const profileDiv = document.getElementById('profile');
The getElementById()
method is straightforward because IDs are unique within an HTML document. There should only ever be one ID per element.
Question 3: What are the differences between querySelector
and querySelectorAll
?
Answer:
querySelector
returns the first element that matches a specified CSS selector.querySelectorAll
returns a static NodeList of all elements that match the specified CSS selector.
const firstItem = document.querySelector('.item');
const allItems = document.querySelectorAll('.item');
Explanation: querySelector
is useful when you need only the first occurrence of an element, while querySelectorAll
is handy for manipulating or iterating over multiple elements.
Question 4: How do you add a new element to the DOM?
Answer: You can add a new element to the DOM using the createElement()
and appendChild()
methods. Here’s an example:
const newDiv = document.createElement('div');
newDiv.textContent = 'Hello, DOM!';
document.body.appendChild(newDiv);
Explanation: The createElement()
method is used to create a new element, and appendChild()
adds the new element to the DOM, making it visible on the page.
Question 5: How can you remove an element from the DOM?
Answer: To remove an element, use the removeChild
method. First, get a reference to the parent element, then call removeChild
:
const parent = document.getElementById('list');
const child = document.getElementById('item');
parent.removeChild(child);
Explanation: Use the removeChild()
method to remove a child element from its parent element. First, obtain a reference to the parent element and the child element you want to remove. Then, call the removeChild
method on the parent element, passing in the child element as an argument. This will remove the child element from the DOM.
Question 6: What is event delegation and how does it work?
Answer: Event delegation involves adding a single event listener to a parent element to manage events for its children. It takes advantage of event bubbling.
document.getElementById('list').addEventListener('click', function(event) {
if (event.target && event.target.matches('li.item')) {
console.log('List item clicked');
}
});
Explanation: Event delegation reduces the number of event listeners needed. Instead of adding an event listener to each child element, you add a single event listener to the parent element. When an event occurs on a child element, it bubbles up to the parent element, allowing the parent element’s event listener to handle the event.
Question 7: How do you change the style of an element using JavaScript?
Answer: You can change an element’s style by modifying its style property, like so:
const box = document.getElementById('box');
box.style.backgroundColor = 'red';
box.style.width = '100px';
box.style.height = '100px';
Explanation: Directly modifying the style properties of an element allows you to dynamically change its appearance using JavaScript. This can be particularly useful in scenarios where you need to provide immediate visual feedback based on user interactions.
You might think about registration fields – if a user is typing in a password that is lacking strength, you could set some paragraph tags to the color red. Else, if the password looks good, set the color to green.
Question 8: What is the difference between innerHTML
and textContent
?
Answer:
- innerHTML sets or gets the HTML or XML markup contained within an element.
- textContent sets or gets the text content of an element and its descendants.
const element = document.getElementById('content');
element.innerHTML = '<strong>Hello, World!</strong>'; // Renders bold text
element.textContent = '<strong>Hello, World!</strong>'; // Renders plain text
Explanation: innerHTML
allows you to insert HTML tags and render them as part of the document, making it useful for dynamically generating HTML content. However, using innerHTML can expose your application to security risks, such as cross-site scripting (XSS) attacks, if the content is not properly sanitized.
textContent
, on the other hand, only sets or retrieves the text content of an element, escaping any HTML tags. This ensures that any HTML markup is treated as plain text and not rendered, making it a safer option for displaying user-generated content or any content that does not require HTML formatting.
Conclusion
Mastering JavaScript DOM manipulation is crucial for any front-end developer. Through this article, we’ve explored some of the top interview questions that test your understanding of the DOM, including techniques for element removal, event delegation, style manipulation, differences between innerHTML
and textContent
, etc.. These concepts are foundational for creating dynamic and interactive web applications.
We hope you enjoyed this article. Feel free to share your thoughts below!
Related Posts
Finding Free and Discounted Programming Books
As an avid reader, I’m always looking for places to find my next book. If they’re free, even better. Although it’s not always so easy finding them, there are plenty available online.
Read moreGetting Started with Google Cloud
In this article, we’re going to be taking a first look at Google Cloud, a leading player in the world of cloud computing, offers services and tools designed to drive innovation and ease operations.
Read moreThe Great JavaScript Debate: To Semicolon or Not?
Since I’ve started learning this language, JavaScript has undergone some heavy changes. Most notably, it seems to be the norm to not use semicolons anymore.
Read more