The addEventListener()
method is a powerful tool in web development that allows developers to add event handlers to an HTML element. It is used to specify a function that will be called when a particular event is fired on that element. In this article, we will explore the addEventListener()
method, how it works, and some of its use cases.
How addEventListener()
works
The addEventListener()
method is used to add an event listener to an HTML element. It takes two arguments: the type of event to listen for and the function to be called when the event is fired.
element.addEventListener(eventType, eventHandlerFunction);
In the code above, element
is the HTML element to which the event listener is to be attached. eventType
is a string that represents the type of event to listen for, such as click, mouseover, or keydown. eventHandlerFunction
is a function that will be called when the specified event is fired.
The addEventListener()
method can be used to attach multiple event listeners to an HTML element, each listening for a different event. If the same event listener function is to be used for multiple events, it is recommended to define the function as a separate variable and pass that variable to the addEventListener()
method for each event.
Advantages of addEventListener()
There are several advantages to using the addEventListener()
method:
- Multiple event handlers can be added to a single HTML element, each listening for a different event.
- Event listeners can be easily removed using the
removeEventListener()
method, which takes the same arguments as theaddEventListener()
method. - The
addEventListener()
method can be used to attach event listeners to any HTML element, including dynamically created elements. - The
addEventListener()
method provides a more efficient way of adding event listeners than using the HTML attribute onclick or other similar attributes.
Use cases for addEventListener()
The addEventListener()
method can be used in many different scenarios. Here are some examples:
- Form validation: When a user submits a form, the submit event is fired. An event listener can be added to the form element to validate the user input before the form is submitted.
- Navigation: When a user clicks on a navigation link, the click event is fired. An event listener can be added to the link element to handle the navigation logic.
- Animations: When an element is hovered over or clicked, the mouseover or click event is fired. An event listener can be added to the element to trigger an animation.
- User input: When a user types in an input field, the keyup event is fired. An event listener can be added to the input element to dynamically update the page based on the user input.
Example of addEventListener()
Let’s take a quick example of using addEventListener()
.
We’ll start with some HTML:
<button id="myButton">Click me</button>
And then add an event listener onto the button element with JavaScript:
const myButton = document.querySelector("#myButton");
function handleClick() {
console.log("Button clicked!");
}
myButton.addEventListener("click", handleClick);
In the example above, we first select the button element using document.querySelector()
, and then define a function called handleClick()
that will be called when the button is clicked. We then add the event listener to the button using addEventListener()
, passing in the string ‘click
’ to indicate that we want to listen for click events, and the handleClick
function as the event handler.
Now, whenever the button is clicked, the handleClick
function will be called, and the message 'Button clicked!'
will be logged to the console.
Conclusion
In conclusion, the addEventListener()
method is a powerful tool that allows developers to add event listeners to HTML elements. It provides a more efficient and flexible way of handling events than using HTML attributes. With its ability to attach multiple event listeners to a single element and its ease of use, addEventListener()
is an essential method for web developers to know and utilize in their projects.
Topics
About the Author
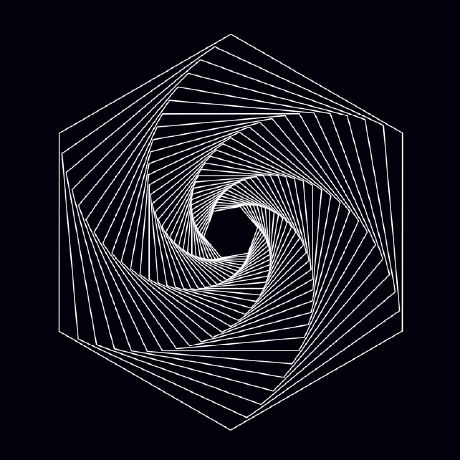
Matt Fay
Matt is the founder of JavaScript Today, a platform dedicated to high-quality JavaScript education and commentary. With a deep curiosity for technology, he gravitates toward understanding how things work and has been diving into the world of information security. Outside of coding, Matt enjoys exploring languages; he can understand some Russian and Italian, and is currently learning Thai.
Discussion (Loading...)
Join the Discussion
Sign in to share your thoughts and engage with the JavaScript Today community.